Introduction:
In the ever-evolving landscape of artificial intelligence, Keras has emerged as one of the most popular and accessible frameworks for building deep learning models. Its simplicity, versatility, and compatibility with powerful backends like TensorFlow make it an excellent choice for both beginners and seasoned developers. This article will explore the key features and advantages of Keras, its role in deep learning, and how to harness its power for cutting-edge AI applications.
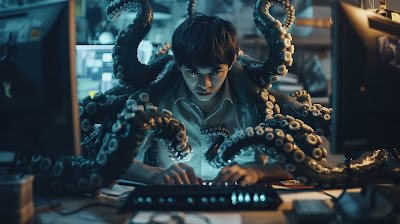
What is Keras?
The Python neural network library Keras is open-source. It offers a sophisticated interface for deep learning model construction and training. Initially developed by François Chollet, Keras is designed to be user-friendly, modular, and extensible, making it ideal for rapid experimentation and prototyping.
One of the standout features of Keras is its ability to run on top of multiple backends, including TensorFlow, Microsoft Cognitive Toolkit (CNTK), and Theano. While TensorFlow has become the dominant backend, Keras abstracts the complexity of these frameworks, allowing developers to focus on building models rather than worrying about the underlying infrastructure.
Why Choose Keras for Deep Learning?
There are several reasons why Keras has become the go-to deep learning framework
for many AI researchers and developers:
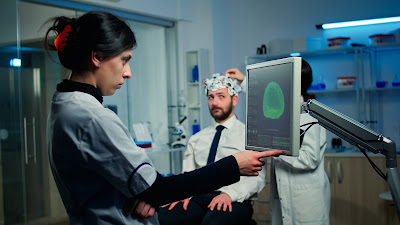
User-Friendly API: Keras provides an easy-to-use API that simplifies the
process of building neural networks. Its intuitive design allows developers to
implement complex models with just a few lines of code.
Modularity: Keras is built with modularity in mind, meaning that you can
easily combine different types of models, layers, and activation functions.
This flexibility is crucial when experimenting with new architectures or
fine-tuning existing ones.
Support for Multiple Backends: Although TensorFlow is the most common
backend, Keras allows you to switch between different computational engines.
This flexibility can be useful when optimizing performance for specific
hardware or cloud environments.
Pre-trained Models: Keras offers a wide range of pre-trained models, such
as VGG16, ResNet, and Inception. These models, trained on large datasets like
ImageNet, can be fine-tuned for specific tasks, reducing the time and resources
required to build new models from scratch.
Scalability: Keras is scalable and can handle everything from small
datasets on a single machine to large-scale, distributed training across
multiple GPUs or even entire cloud infrastructures.
Community Support: Keras has a large and active community of developers and
researchers contributing to its development. This means that you can find
extensive documentation, tutorials, and third-party libraries to help you
overcome any challenges you encounter.
Key Features of Keras:
Let's dive deeper into some of the standout features that make Keras a powerful tool for deep learning:
1. Sequential and Functional APIs:
The Sequential API and the Functional API are the two primary methods for
creating neural networks that Keras provides.
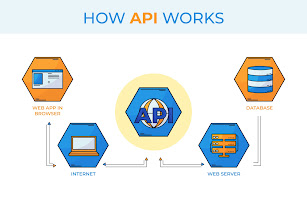
Sequential API: This is the simplest way to build a model in Keras. You
create a linear stack of layers where each layer has only one input and one
output. This API is ideal for straightforward models like feedforward neural
networks.
python
Copy
from keras.models import Sequential
from keras.layers import Dense
model = Sequential()
model.add(Dense(128, activation='relu', input_shape=(784,)))
model.add(Dense(10, activation='softmax'))
Functional API: For more complex models, such as those with multiple inputs
and outputs or shared layers, the Functional API is the better option. It
provides more flexibility and is recommended for advanced architectures like
residual networks and attention mechanisms.
python
Copy
from keras.models import Model
from keras.layers import Input, Dense
inputs = Input(shape=(784,))
x = Dense(128, activation='relu')(inputs)
outputs = Dense(10, activation='softmax')(x)
model = Model(inputs, outputs)
2. Layers and Activation Functions:
Keras provides a wide variety of layers, including:
Dense: Fully connected layers used in feedforward neural networks.
Conv2D: Convolutional layers for image processing.
LSTM: Long Short-Term Memory layers for sequence data like time series or
text.
Dropout: Regularization layers to prevent overfitting by randomly dropping
neurons during training.
In addition, Keras supports a variety of activation functions, such as ReLU, sigmoid, softmax, and tanh, allowing you to experiment with different approaches to learning and classification.
3. Optimizers and Loss Functions:
Keras supports a variety of optimizers, including SGD, Adam, RMSprop, and more. These optimizers adjust the learning rate during training, helping the model converge faster. Keras also offers several loss functions based on the type of task:
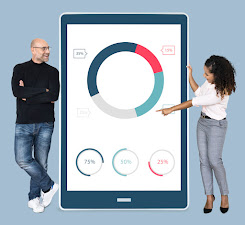
Categorical Crossentropy: Used for multi-class classification tasks.
Binary Crossentropy: Used for binary classification.
For regression tasks, mean squared error, or MSE, is frequently utilized.
4. Callbacks and Customizations:
Keras allows you to leverage callbacks during training, which can be used to monitor performance, adjust learning rates, or save checkpoints. Some common callbacks include:
EarlyStopping: Stops training when performance stops improving.
ModelCheckpoint: Saves the model after every epoch if the validation
accuracy improves.
LearningRateScheduler: Dynamically adjusts the learning rate during
training.
You can also define custom layers, loss functions, and optimizers if the built-in options do not meet your specific needs.
Building a Deep Learning Model with Keras:
Let’s walk through a simple example of building and training a deep learning model using Keras. We'll create a model to classify digits from the MNIST dataset, a popular dataset in the field of machine learning.
Step 1: Import Libraries and Load Data:
First, we need to import the necessary libraries and load the MNIST dataset, which contains 28x28 grayscale images of handwritten digits.
python
Copy
import keras
from keras.datasets import mnist
from keras.models import Sequential
from keras.layers import Dense, Flatten
from keras.utils import to_categorical
# Load the MNIST dataset
(x_train, y_train), (x_test, y_test) = mnist.load_data()
# Normalize the pixel values to be between 0 and 1
x_train = x_train / 255.0
x_test = x_test / 255.0
# One-hot encode the labels
y_train = to_categorical(y_train, 10)
y_test = to_categorical(y_test, 10)
Step 2: Build the Model:
Next, we'll construct a simple feedforward neural network using the Sequential API.
python
Copy
model = Sequential()
model.add(Flatten(input_shape=(28, 28)))
model.add(Dense(128, activation='relu'))
model.add(Dense(10, activation='softmax'))
Step 3: Compile the Model:
We will use categorical crossentropy as our loss function (since this is a multi-class classification problem) and Adam as the optimizer.
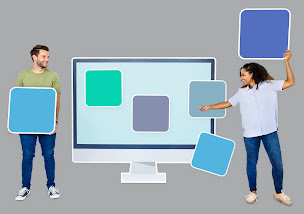
python
Copy
model.compile(optimizer='adam',
loss='categorical_crossentropy',
metrics=['accuracy'])
Step 4: Train the Model:
Now, we can train the model with the training data. We’ll also use the test data as validation during training.
python
Copy
model.fit(x_train, y_train, epochs=5, batch_size=32,
validation_data=(x_test, y_test))
Step 5: Evaluate the Model:
Lastly, we assess how well the model performs on the test set.
python
Copy
test_loss, test_acc = model.evaluate(x_test, y_test)
print(f'Test accuracy: {test_acc}')
Advanced Topics: Transfer Learning with Keras:
One of the most exciting applications of Keras is transfer learning, where you take a pre-trained model and fine-tune it for a new task. This is particularly useful when you have limited data for your specific problem but can leverage large datasets that have already been used to train existing models.
For example, you could use a pre-trained model like VGG16 or ResNet for image classification tasks. These models are available in Keras through the keras.applications module.
python
Copy
from keras. applications import VGG16
# Load the ImageNet-pre-trained VGG16 model.
vgg_model = VGG16(weights='imagenet', include_top=False, input_shape=(224,
224, 3))
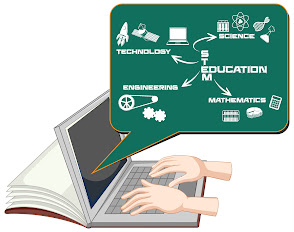
Conclusion:
Keras is an incredibly powerful and flexible framework that makes building and training deep learning models accessible to both beginners and experts. With its intuitive API, support for multiple backends, and extensive community resources, Keras is the perfect tool for anyone looking to dive into the world of AI and deep learning.
Whether you're working on simple feedforward networks or advanced models like convolutional neural networks (CNNs) and recurrent networks (RNNs), Keras provides the tools you need to succeed. By leveraging its features and capabilities, you can build state-of-the-art models and contribute to the future of AI research.
With its ease of use and powerful features, Keras continues to be a leading
choice for AI developers worldwide.
0 Comments